Notifications with a Webex Bot
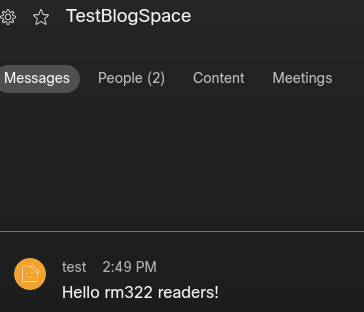
In studying for Cisco’s 350-901 DEVCOR Exam, I wanted to make sure I got some hands on practice with some of the objectives. Topic 3.1’s, objectives is: Construct API requests to implement chatops with Webex Teams API. I decided to look into creating a bot that would notify me about certain things going on around my house or happening on my network. Webex’s API is real easy to use and Cisco provides some great documentation on their site. The documentation might be intimidating to some, so this will serve a quick down and dirty demo.
First things first…go ahead and create a free webex account. Once you have an account, you will need to create a bot at the developer page. In the upper right corner click your initials and select “My Webex Apps”
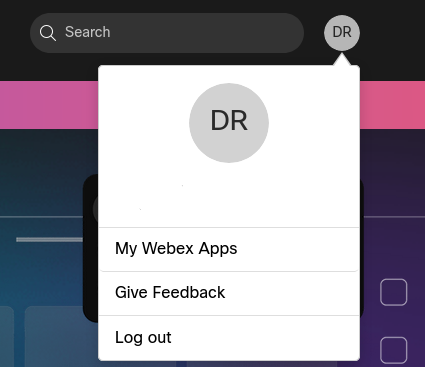
You will be taken to the New App page. Select the “Create a Bot” button. The first page involved in creating a bot involves giving it a name and description. Webex provides a detailed description for every choice you make here. Once you have filled in the information click the “Add Bot” near the bottom of the page.
You will be presented with an access token and a Bot ID. While I haven’t found a use for the Bot ID the access token will be used to authenticate your bot later. Go ahead and copy this token and place it into an empty file. Don’t worry if you lose your access token, you can regenerate one later if need be.

In order for your bot to participate in any chat with you will have to add it to a space/room on Webex. Below I am adding the bot I just created. You will have to press the “invite” button, followed by the “add” button. Once this is done you should see the bot in the recently created space.
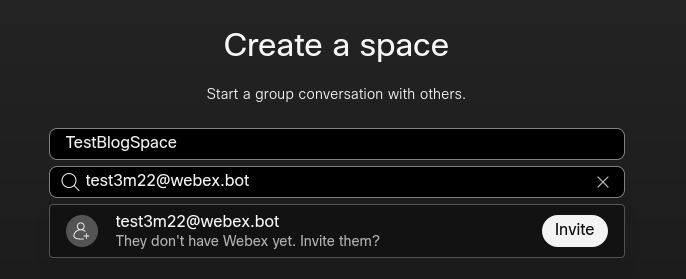
Before the bot can send messages in this space, it has to find a way to identify the room. Cisco provides an API call that will list the rooms the bot is participating in. Since we’ve only added the bot to one room this should be pretty easy. To get the room we will use the python requests module, create a few headers, then submit a query to the webex API. Below is an easy method to do this:
import requests
# Bot Information
botToken = 'M2QwZWM2NTEtYzk0Zi00MmQzLTkyNzMtNjM4ZTZmOTYwMDRhY2I4YjllNDMtNDVk_PF84_3f179706-f11a-4ab7-ba3e-thisisfake'
# API information along with the required Authorization header
url = 'https://api.ciscospark.com/v1/'
headers = {'Authorization': 'Bearer ' + botToken}
# Send message to API and print the room ID
getRooms = requests.get(url + 'rooms', headers=headers).json()
print(getRooms['items'][0]['id'])

We will now take the Room ID and change our code to submit a message instead of requesting a list of rooms. Below is a short example of how easy it is to send a message:
import requests
message = input("What do you want to say? ")
# Bot Information
botToken = 'M2QwZWM2NTEtYzk0Zi00MmQzLTkyNzMtNjM4ZTZmOTYwMDRhY2I4YjllNDMtNDVk_PF84_3f179706-f11a-4ab7-ba3e-thisisfake'
roomId = 'Y2lzY29zcGFyazovL3VzL1JPT00vYWM5YTAwYjAtY2IwZC0xMWVjLTk3MDAtYzUxYmJkNGRhNGIy'
# API information along with the required Authorization header
url = 'https://api.ciscospark.com/v1/'
headers = {'Authorization': 'Bearer ' + botToken}
# Message along with the room we want it posted in
postData = {'roomId': roomId,
'text': message}
# Post a message to the selected room
postMessage = requests.post(url + 'messages', json=postData, headers=headers).json()
Notice that we are now submitting a POST instead of a GET. If you are using the example I provided above your command and output might look like this:

If you are in Webex you should have received a message. Here is the message I just posted using the above command:
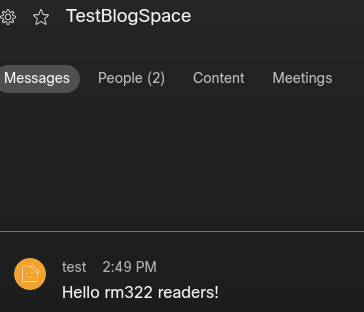
Now that you have a bot you can tidy up the above message script to submit more important messages. In my case I changed my code into a function and allow other processes to use it to send me updates like when a VPN user connects to my network, when my public IP changes (see last post), or daily firewall block statistics.
Webex makes creating a bot and sending messages very easy with its API. You will need a basic understanding of python and working with APIs to get your bot off the ground. However, this is a good project that anyone interested in working with APIs can look to start with.