Migrating/Updating my TinyDB MAC API – FastAPI Setup

Now that we have the ability to interact with our database, its time to explore how we can use our API to interact with the queries we made. In this post, I’ll show how to pull a list of the mac addresses currently on my network, followed by pulling information about a specific mac address. I primarily used FastAPI’s guides to help get me started, then tinkered until I had what I was looking for.
The first API call I was most interested in was pulling what mac addresses were currently on my network. In my case, I decided that the URI “current_macs” would work best for this task. Since I constructed a SQL query that exactly matched this property, my code was relatively simple…just reference crud’s select_recent_macs function.
@app.get("/current_macs/")
# List all macs that were connected at the last queried time
async def select_current_macs(db: Session = Depends(get_db)):
recent_macs = crud.select_recent_macs(db)
return recent_macs
Now by either curling (shown in my last post) or visiting the “current_macs” URI, a json formatted list would be returned:
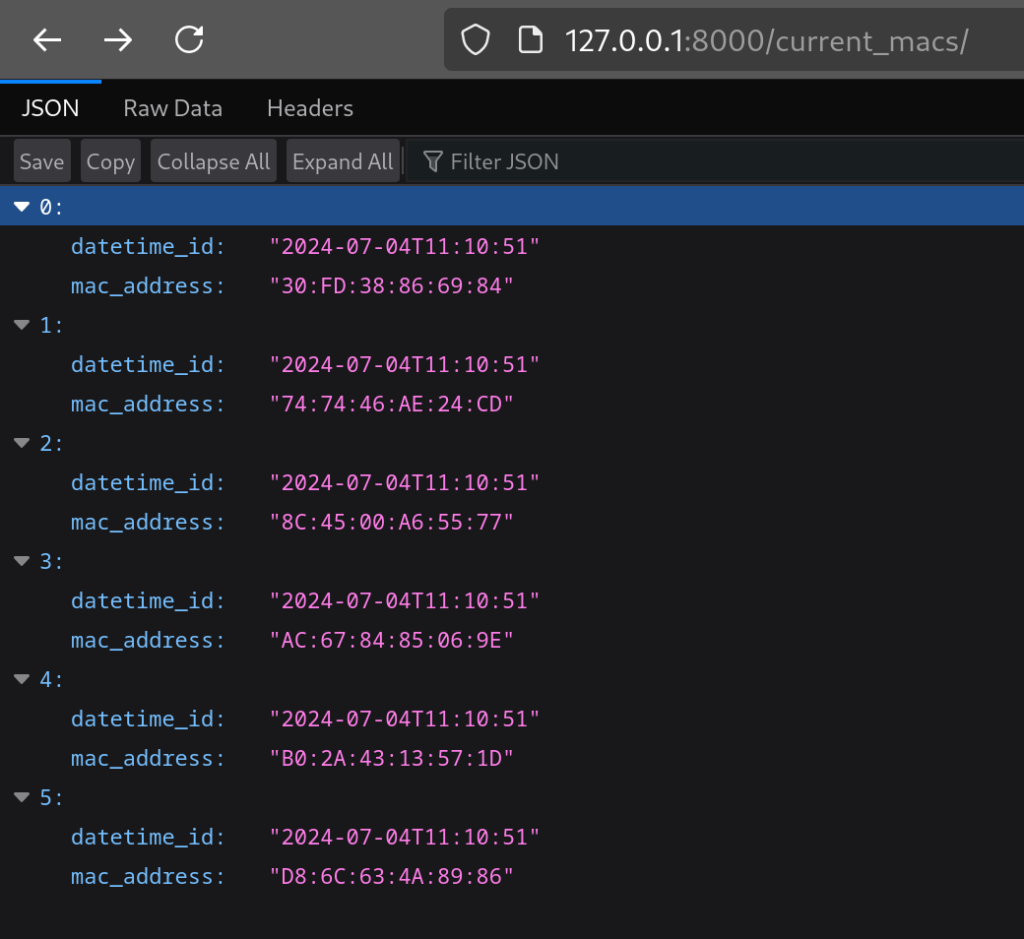
I also wanted the ability to be able to look at a specific mac address. In the case of this URI, I would have to provide the mac address to the API so that it could provide that specific lookup. To support this I created the following:
@app.get("/known_macs/{mac_address}", response_model=schemas.MacAddress)
# Get information about a specific mac address
async def select_indiv_known_mac(mac_address: str, db: Session = Depends(get_db)):
mac_address_info = crud.select_indiv_known_mac(db, mac_address=mac_address)
if mac_address_info is None:
raise HTTPException(status_code=404, detail="MAC Address not found in database")
else:
return mac_address_info
Once again I specify a URI, but this time I include the mac address as a variable URI endpoint. I created another SQL query that would take filter based on the specific mac address and return info called “selected_indiv_known_mac” for this purpose. Finally, I either return a 404 error if the mac address is not found in my database, or I return the mac’s information if it does.
